I have done the most basic Admin Grid Using Layout in Previous post so I will introduce how How to add/update item in Magento backend . I hope that after reading the post and other posts in the Tutorial, You can easy to make functions to Manager items and database . The Post include 2 parts:
- Create form add/update
- Create Controller Saving data.
1. Create from add/update
- Create Controller app/code/Magebay/Hello/Controller/Adminhtml/Posts/Edit.php
<?php namespace Magebay\Hello\Controller\Adminhtml\Posts; use Magebay\Hello\Controller\Adminhtml\Posts; use Magebay\Hello\Model\PostsFactory; use Magento\Backend\App\Action\Context; use Magento\Framework\Registry; use Magento\Framework\View\Result\PageFactory; class Edit extends Posts { protected $resPostFactory; public function __construct( Context $context, Registry $coreRegistry, PageFactory $resultPageFactory, PostsFactory $postsFactory, \Magebay\Hello\Model\ResourceModel\PostsFactory $resPostFactory ) { parent::__construct($context, $coreRegistry, $resultPageFactory, $postsFactory); $this->resPostFactory = $resPostFactory; } /** * @return void */ public function execute() { $postId = $this->getRequest()->getParam('id'); $model = $this->postsFactory->create(); $resModel = $this->resPostFactory->create(); if ($postId) { $resModel->load($model,$postId); if (!$model->getId()) { $this->messageManager->addError(__('This news no longer exists.')); $this->_redirect('*/*/'); return; } } // Restore previously entered form data from session $data = $this->_session->getPostData(true); if (!empty($data)) { $model->setData($data); } $this->coreRegistry->register('post_data', $model); $resultPage = $this->resultPageFactory->create(); $resultPage->setActiveMenu('Magebay_Hello::hello_menu'); $resultPage->getConfig()->getTitle()->prepend(__('Manager Post')); return $resultPage; } }
- Create file app/code/Magebay/Hello/Block/Adminhtml/Posts/Edit.php
<?php namespace Magebay\Hello\Block\Adminhtml\Posts; use Magento\Backend\Block\Widget\Form\Container; use Magento\Backend\Block\Widget\Context; use Magento\Framework\Registry; class Edit extends Container { /** * Core registry * * @var \Magento\Framework\Registry */ protected $_coreRegistry = null; /** * @param Context $context * @param Registry $registry * @param array $data */ public function __construct( Context $context, Registry $registry, array $data = [] ) { $this->_coreRegistry = $registry; parent::__construct($context, $data); } /** * Class constructor * * @return void */ protected function _construct() { $this->_objectId = 'news_id'; $this->_controller = 'adminhtml_posts'; $this->_blockGroup = 'Magebay_Hello'; parent::_construct(); $this->buttonList->update('save', 'label', __('Save')); $this->buttonList->add( 'saveandcontinue', [ 'label' => __('Save and Continue Edit'), 'class' => 'save', 'data_attribute' => [ 'mage-init' => [ 'button' => [ 'event' => 'saveAndContinueEdit', 'target' => '#edit_form' ] ] ] ], -100 ); $this->buttonList->update('delete', 'label', __('Delete')); } /** * Retrieve text for header element depending on loaded news * * @return string */ public function getHeaderText() { $post = $this->_coreRegistry->registry('post_data'); if ($post->getId()) { $postTitle = $this->escapeHtml($post->getTitle()); return __("Edit News '%1'", $postTitle); } else { return __('Add News'); } } /** * Prepare layout * * @return \Magento\Framework\View\Element\AbstractBlock */ protected function _prepareLayout() { $this->_formScripts[] = " function toggleEditor() { if (tinyMCE.getInstanceById('post_content') == null) { tinyMCE.execCommand('mceAddControl', false, 'post_content'); } else { tinyMCE.execCommand('mceRemoveControl', false, 'post_content'); } }; "; return parent::_prepareLayout(); } }
- Create file app/code/Magebay/Hello/Block/Adminhtml/Posts/Edit/Tabs.php
<?php namespace Magebay\Hello\Block\Adminhtml\Posts\Edit; use Magento\Backend\Block\Widget\Tabs as WidgetTabs; class Tabs extends WidgetTabs { /** * Class constructor * * @return void */ protected function _construct() { parent::_construct(); $this->setId('post_edit_tabs'); $this->setDestElementId('edit_form'); $this->setTitle(__('Post Information')); } /** * @return $this */ protected function _beforeToHtml() { $this->addTab( 'post_info', [ 'label' => __('General'), 'title' => __('General'), 'content' => $this->getLayout()->createBlock( 'Magebay\Hello\Block\Adminhtml\Posts\Edit\Tab\Info' )->toHtml(), 'active' => true ] ); return parent::_beforeToHtml(); } }
- Create file
app/code/Magebay/Hello/Block/Adminhtml/Posts/Edit/Form.php
<?php namespace Magebay\Hello\Block\Adminhtml\Posts\Edit; use Magento\Backend\Block\Widget\Tabs as WidgetTabs; class Tabs extends WidgetTabs { /** * Class constructor * * @return void */ protected function _construct() { parent::_construct(); $this->setId('post_edit_tabs'); $this->setDestElementId('edit_form'); $this->setTitle(__('Post Information')); } /** * @return $this */ protected function _beforeToHtml() { $this->addTab( 'post_info', [ 'label' => __('General'), 'title' => __('General'), 'content' => $this->getLayout()->createBlock( 'Magebay\Hello\Block\Adminhtml\Posts\Edit\Tab\Info' )->toHtml(), 'active' => true ] ); return parent::_beforeToHtml(); } }
- Create file
app/code/Magebay/Hello/Block/Adminhtml/Posts/Edit/Tab/Info.php
<?php namespace Magebay\Hello\Block\Adminhtml\Posts\Edit\Tab; use Magento\Backend\Block\Widget\Form\Generic; use Magento\Backend\Block\Widget\Tab\TabInterface; use Magento\Backend\Block\Template\Context; use Magento\Framework\Registry; use Magento\Framework\Data\FormFactory; use Magento\Cms\Model\Wysiwyg\Config; use Magebay\Hello\Model\System\Config\Status; class Info extends Generic implements TabInterface { /** * @var \Magento\Cms\Model\Wysiwyg\Config */ protected $_wysiwygConfig; /** * @var \Magebay\Hello\Model\System\Config\Status */ protected $_status; /** * @param Context $context * @param Registry $registry * @param FormFactory $formFactory * @param Config $wysiwygConfig * @param Status $status * @param array $data */ public function __construct( Context $context, Registry $registry, FormFactory $formFactory, Config $wysiwygConfig, Status $status, array $data = [] ) { $this->_wysiwygConfig = $wysiwygConfig; $this->_status = $status; parent::__construct($context, $registry, $formFactory, $data); } /** * Prepare form fields * * @return \Magento\Backend\Block\Widget\Form */ protected function _prepareForm() { /** @var $model \Magebay\Hello\Model\PostsFactory */ $model = $this->_coreRegistry->registry('post_data'); /** @var \Magento\Framework\Data\Form $form */ $form = $this->_formFactory->create(); $form->setHtmlIdPrefix('posts_'); $form->setFieldNameSuffix('posts'); $fieldset = $form->addFieldset( 'base_fieldset', ['legend' => __('General')] ); if ($model->getId()) { $fieldset->addField( 'news_id', 'hidden', ['name' => 'news_id'] ); } $fieldset->addField( 'title', 'text', [ 'name' => 'title', 'label' => __('Title') ] ); $fieldset->addField( 'status', 'select', [ 'name' => 'status', 'label' => __('Status'), 'options' => $this->_status->toOptionArray() ] ); $fieldset->addField( 'position', 'text', [ 'name' => 'position', 'label' => __('Position') ] ); $fieldset->addField('description', 'editor', [ 'name' => 'description', 'label' => 'Description', 'config' => $this->_wysiwygConfig->getConfig(), 'wysiwyg' => true, 'required' => false ]); $data = $model->getData(); $form->setValues($data); $this->setForm($form); return parent::_prepareForm(); } /** * Prepare label for tab * * @return string */ public function getTabLabel() { return __('News Info'); } /** * Prepare title for tab * * @return string */ public function getTabTitle() { return __('News Info'); } /** * {@inheritdoc} */ public function canShowTab() { return true; } /** * {@inheritdoc} */ public function isHidden() { return false; } }
Now , clear cache and click new post or Edit link in item, you will get form add/update item like screen image
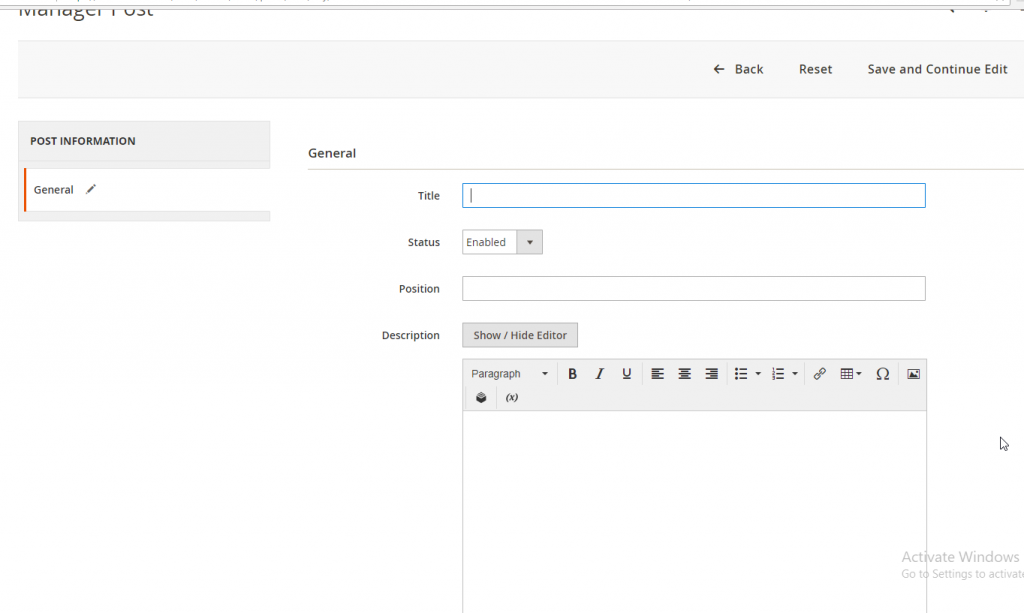
2. Create Controller saving data
- Create file save in app/code/Magebay/Hello/Controller/Adminhtml/Posts/
<?php namespace Magebay\Hello\Controller\Adminhtml\Posts; use Magebay\Hello\Controller\Adminhtml\Posts; use Magebay\Hello\Model\PostsFactory; use Magento\Backend\App\Action\Context; use Magento\Framework\Registry; use Magento\Framework\View\Result\PageFactory; class Save extends Posts { protected $resPostFactory; public function __construct( Context $context, Registry $coreRegistry, PageFactory $resultPageFactory, PostsFactory $postsFactory, \Magebay\Hello\Model\ResourceModel\PostsFactory $resPostFactory ) { parent::__construct($context, $coreRegistry, $resultPageFactory, $postsFactory); $this->resPostFactory = $resPostFactory; } /** * @return void */ public function execute() { $isPost = $this->getRequest()->getPost(); if($isPost) { $formData = $this->getRequest()->getParam('posts'); $postId = isset($formData['news_id']) ? (int)$formData['news_id'] : 0; if($postId == 0) unset($formData['news_id']); try { $model = $this->postsFactory->create(); $resModel = $this->resPostFactory->create(); $model->setData($formData); $resModel->save($model); if($postId == 0) $message = __('The post was added successfully!'); else $message = __('The post was update successfully!'); $this->messageManager->addSuccessMessage($message); if ($this->getRequest()->getParam('back')) { $this->_redirect('*/*/edit', ['id' => $model->getId(), '_current' => true]); return; } $this->_redirect('*/*/'); return; } catch (\Exception $exception) { $this->messageManager->addErrorMessage($exception->getMessage()); } $this->_getSession()->setFormData($formData); $this->_redirect('*/*/edit', ['id' => $vendorId]); } } }
You have done task , you can clear cache and reload form update , you can enter data and save now.
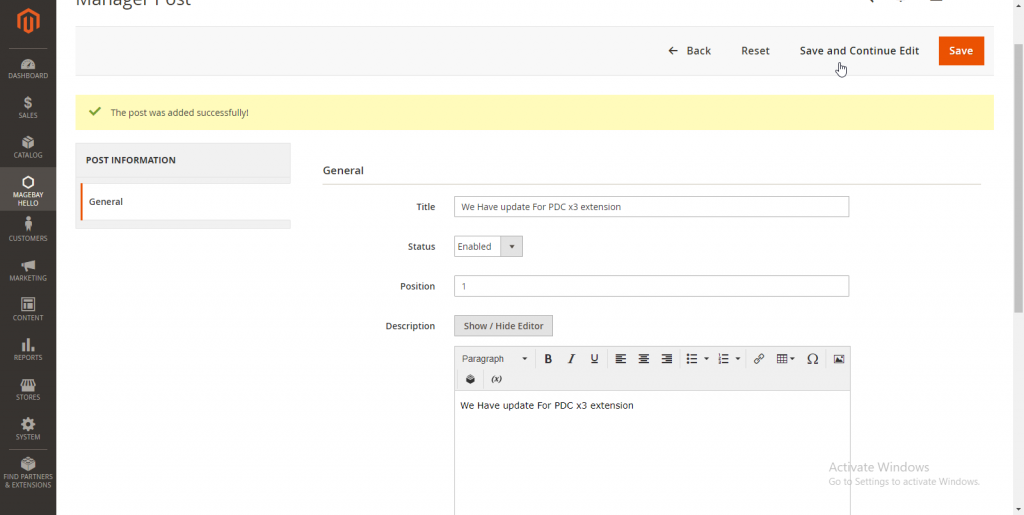
Thank for reading, I hope the post How to add/update item in Magento backend help you understand more about edit/update item in backend because it is importance part in a Magento 2 extension. As you can see, We have done the most issues of extension in backend. I will introduce how to get items to frontend in the next post.