I have introduced How to use Model in previous post, To continue Tutorial, I will introduce How to use Helper and setting in Magento 2 extension . Helper and Setting is very importance for developer when building extension. it help he build helper function and make setting for extension.
1. Use Helper
2 Use Setting
1. Helper
Sometime you have to build the function without get database so he should not use Model because it should be used for database . Helper is the solution best for you in this case. To create Helper, you can Create file Data.php in app/code/Magebay/Hello/Helper/
<?php namespace Magebay\Hello\Helper; use Magento\Framework\App\Helper\AbstractHelper; use Magento\Framework\App\Helper\Context; /** * Class Data * @package Magebay\Hello\Helper */ class Data extends AbstractHelper { /** * Data constructor. * @param Context $context */ public function __construct(Context $context) { parent::__construct($context); } }
Now, I want to have check date function , I will write function to Magebay\Hello\Helper\Data Class
<?php namespace Magebay\Hello\Helper; use Magento\Framework\App\Helper\AbstractHelper; use Magento\Framework\App\Helper\Context; /** * Class Data * @package Magebay\Hello\Helper */ class Data extends AbstractHelper { // .. old code /** * @param string $date * @return bool */ function checkDate($date = '') { $ok = false; if($date != '') { $day = date('w', strtotime($date)); if($day == 0) $ok = true; } return $ok; } }
You can test it in index controller , you can edit file app/code/Magebay/Controller/index/index.php
<?php namespace Magebay\Hello\Controller\Index; use Magento\Framework\App\Action\Context; use Magento\Framework\View\Result\PageFactory; /** * Class Index * @package Magebay\Hello\Controller\Index */ class Index extends \Magento\Framework\App\Action\Action { //..old code /** * @var \Magebay\Hello\Helper\Data */ protected $helloHelper; /** * Index constructor. * @param Context $context * @param PageFactory $resultPageFactory * @param \Magebay\Hello\Model\ResourceModel\Posts\CollectionFactory $postsFactory * @param \Magebay\Hello\Helper\Data $helloHelper */ public function __construct( //..old code \Magebay\Hello\Helper\Data $helloHelper ) { //...old code $this->helloHelper = $helloHelper; } /** * @return \Magento\Framework\App\ResponseInterface|\Magento\Framework\Controller\ResultInterface|void */ public function execute() { //..old code echo "==========Check date, helper function ======== <br>"; $date = '2019-01-20'; if($this->helloHelper->checkDate($date)) { echo "Yes, {$date} is Sunday , I can go to your home"; } else { echo "Yes, {$date} is not Sunday , I was to busy"; } } }
Remove all file in generated/code/Magebay * and reload yourdomain.com/hello/index/index

2. Setting
Setting is very importance in an extension because it help admin can configure data for extension. To create setting, you can create file system.xml in app/code/Magebay/Hello/etc/adminhtml/
<?xml version="1.0"?> <config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="../../../Backend/etc/system_file.xsd"> <system> <tab id="magebay_hello" translate="label" sortOrder="1"> <label>Magebay.com</label> </tab> <section id="blog" translate="label" sortOrder="1" showInDefault="1" showInWebsite="1" showInStore="1"> <label>Magebay Hello</label> <tab>magebay_hello</tab> <resource>Magebay_Hello::system_config</resource> <group id="setting" translate="label" type="text" sortOrder="2" showInDefault="1" showInWebsite="1" showInStore="1"> <label>General Settings</label> <field id="enable" translate="label" type="select" sortOrder="2" showInDefault="1" showInWebsite="1" showInStore="1"> <label>Enable</label> <source_model>Magento\Config\Model\Config\Source\Yesno</source_model> </field> <field id="number_posts" translate="label comment" type="text" sortOrder="5" showInDefault="1" showInWebsite="1" showInStore="1"> <label>Number Posts</label> <comment>Number Posts</comment> <validate>required-entry</validate> </field> </group> </section> </system> </config>
Go To Admin->Stores->Configuration->Magebay.com->Magebay Hello enter configuration and save .
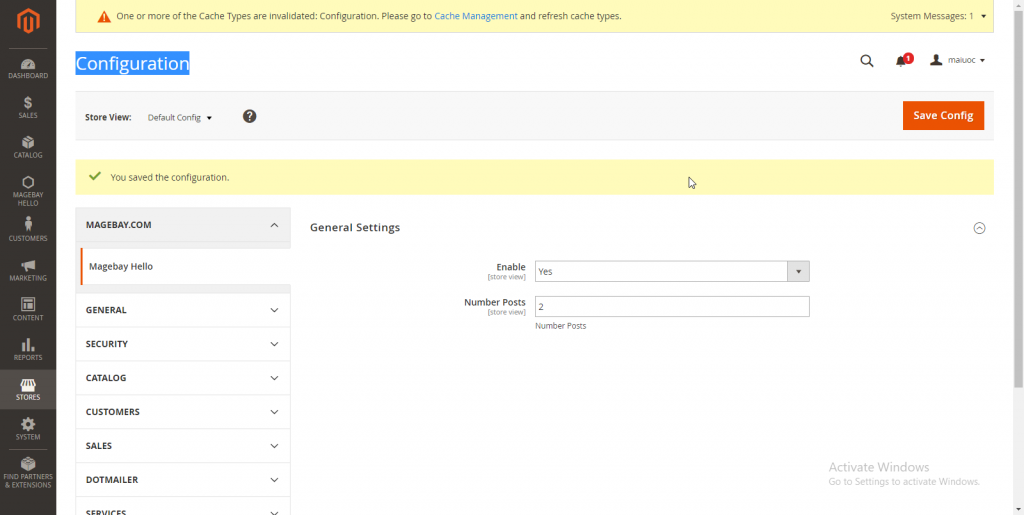
Get it in frontend, You can can it everywhere in frontend but it should make a function in helper and call it . Edit file app/code/Magebay/Hello/Helper/Data.php
<?php namespace Magebay\Hello\Helper; //.. old code use Magento\Store\Model\ScopeInterface; /** * Class Data * @package Magebay\Hello\Helper */ class Data extends AbstractHelper { //.. old code ... /** * @param string $path * @return mixed */ function getHelloSetting($path = '') { return $this->scopeConfig->getValue($path,ScopeInterface::SCOPE_STORE); } }
And call it in Controller by Helper function so you can edit file
app/code/Magebay/Controller/index/index.php
<?php namespace Magebay\Hello\Controller\Index; // ..old code class Index extends \Magento\Framework\App\Action\Action { // ..old code public function execute() { echo "Test Get Data <br>"; $numberPosts = $this->helloHelper->getHelloSetting('blog/setting/number_posts'); echo "Number Posts = {$numberPosts}"; $this->postsFactory->create(); $collection = $this->postsFactory->create() ->addFieldToSelect(array('title','created_at','status','position')) // fields to select ->addFieldToFilter('status',1) // filter status = 1 ->setPageSize($numberPosts) // get 2 items ->setOrder('position','ASC'); // order by position echo '<pre>'; print_r($collection->getData()); echo '<pre>'; //..old code ... } }
As you can see, configuration was called to controller.
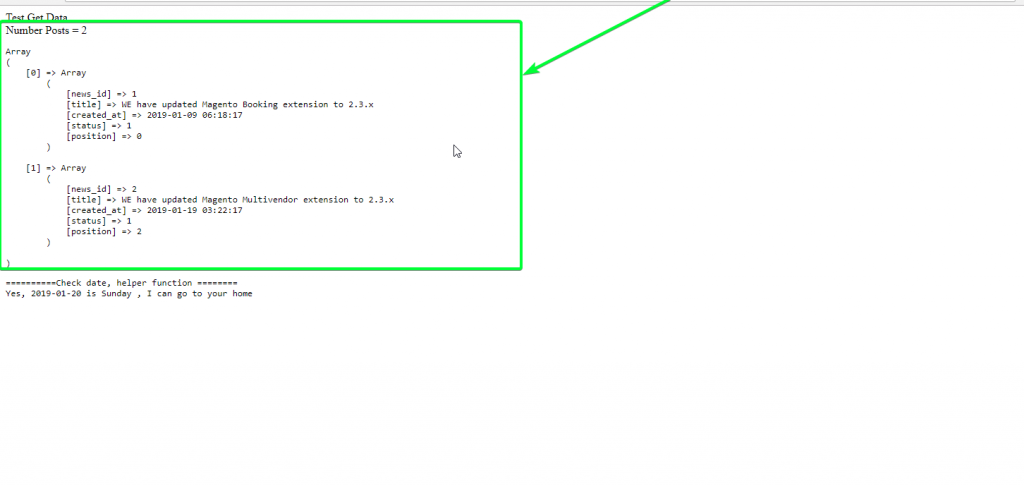
Thank for reading the post , As you can see Helper and Setting is very useful for developer when building extension. you can comment under this post if you have any question. I will introduce How to use event in next post.